How we’ve built our virtual currency on Slack
[This post was originally published on our old blog a few months ago. This is an updated version. Open source code is at the end of the article.]
This is the story of how we’ve built a virtual currency for our teams.
And how we use it today
Everything started around a dinner table. Didier, our Creative Partner, and Thibaud, our co-founder, were discussing the importance of giving “Thanks” to your co-workers, and how it can change the way you enjoy your work on a daily basis.
In our startup studio, we have built 9 startups as of today, which means 150 people throughout the world (and more coming up). It is a great reservoir of talents and offers great opportunities for collaboration and experience sharing. It also means that maintaining healthy ties among all of the teams is a challenge. We’ve already built a set of tools and a mindset called the Network that enables us to keep in touch and communicate real time through Slack.
Yet, it felt like saying ‘thanks for you help’ the “regular” way wasn’t enough. Not for startups. Not for people who do business. It all became clear when we got the check at the end of dinner and Thibaud decided to pay for dinner to thank us for our good work: we would build a new way of saying ‘thanks’ in our community.
We invented a virtual currency to exchange rewards, thankyous and congrats with your colleagues.
It’s called “The Briqs”.
Why a currency?
First and foremost, because it’s fun. It’s no coincidence if one of children’s favorite game is playing shop.
The second reason is that, in a startup, everyone shall have a sense of business: the Briqs appeal to each of our inner Warren Buffet.
Finally, it was a cool tool to build, on technologies we love: Slack’s API — our communication system — and Meteor — a JS framework.
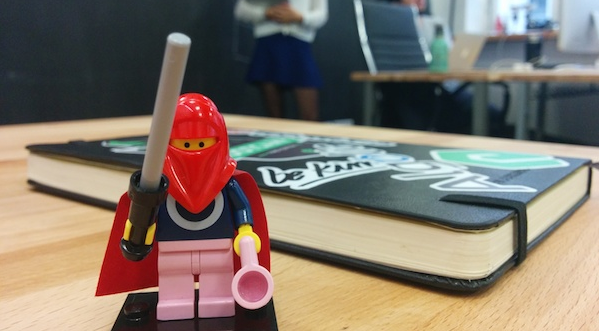
We called our currency “briqs” because we are startup builders. And also, because we love Lego.
How do the Briqs work?
The Briqs have specific characteristic as a monetary system.
The Banq releases briqs
Once you’re part of The Network (that is to say, eFounders and all the startups we’ve built: Textmaster, Mention, Front, Aircall, illustrio, Hivy, and the stealth project), you get 1 briq every 4 hours (that is to say: 42 a week. Yes, that is the number), no matter what. The Banq acts as a central bank, except there is no intermediate banks nor an interest rate or inflation: the “money” emission is regular.
“The regular emission of briqs to members of the network shall not be mistaken for a minimum wage: our talents are so great, it is only a way to fuel production of ideas and execution”. Thibaud Elzière, co-founder
Briqs to trade and briqs to keep
To maintain our currency fluid, we’ve built Banq with specific characteristics.
Watch out for some economical theory here:
As the great economist J.M. Keynes pointed out in his General Theory, money can be either perceived as a means of trade or a means of savings. Indeed, even if briqs do not have an interest rate, you can either use them to trade rewards, but also save them to buy actual goods.
To make sure there is no hoarding inside Banq, we created 2 types of briqs:
- those you get automatically by the Banq: you are bound to give them to other people. In light of the quantity theory of money, economic agents find there is a greater satisfaction (or “utility”) in using your money for consumption than savings. We do too! Just to make sure that people don’t just store these briqs, the total amount of briqs you get is capped at 42.
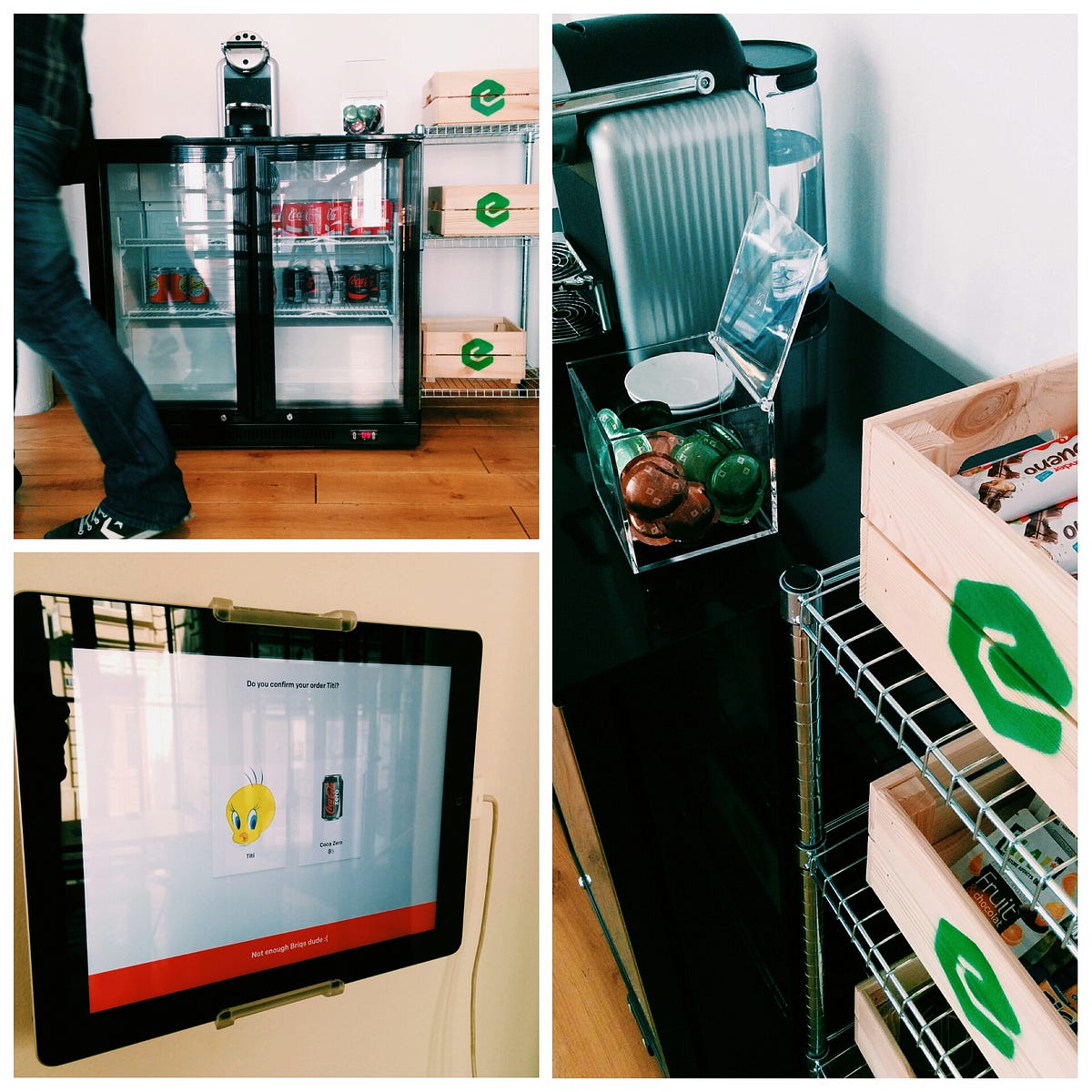
- those someone gives you: you are bound to spend them. We have opened an online shop where you can get books, iPads, Kindle, drinks. In ouf office, Snacks can be bought with Briqs as well.
Then we added a few incentives to earn briqs:
- those you get from referring an employee: see our dedicated article “Project H.I.R.E”
- those you get from spreading news on Twitter: we’ve built another Slack integration to let you retweet stuff easily (the faster, the more briqs)
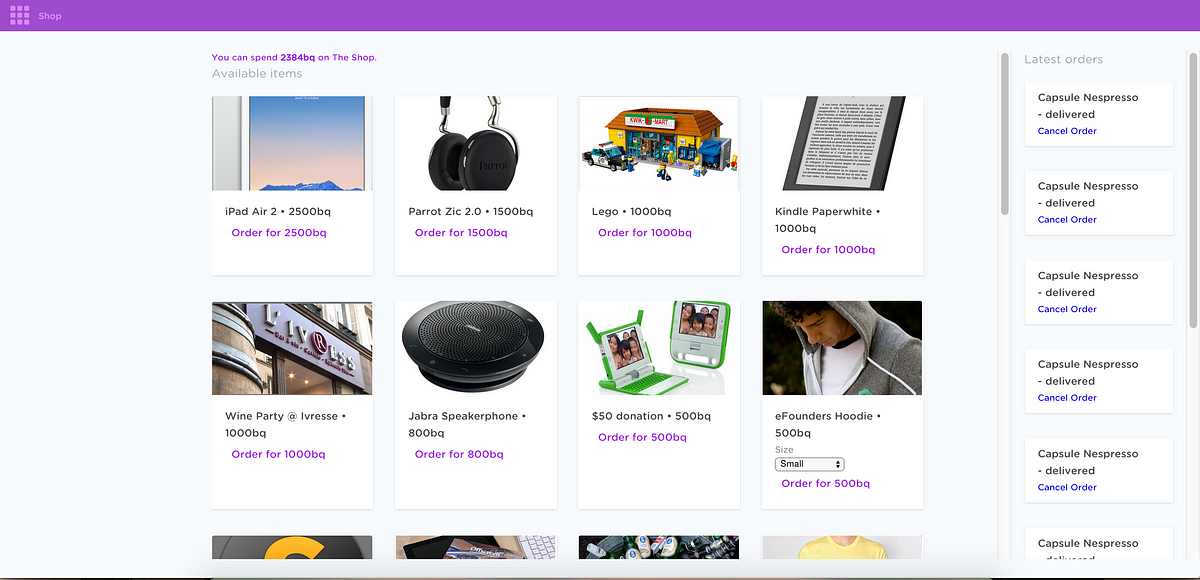
Trading briqs using Slack commands
You give and receive Briqs inside Slack, our communication system. Slack is a really cool collaborative chat used by many startups and companies around the world. You just need to type a command do interact with briqs.
Here are some Slack commands we have added to interact with Banq:
- /briq : display how many briqs you have and can give
- /briq philibert : display how many briqs Philibert have and can give
- /give philibert 1 : give 1bq to Philibert
- /give philibert 100 for the help on design : give 100bq to Philibert and add a purpose of the gift
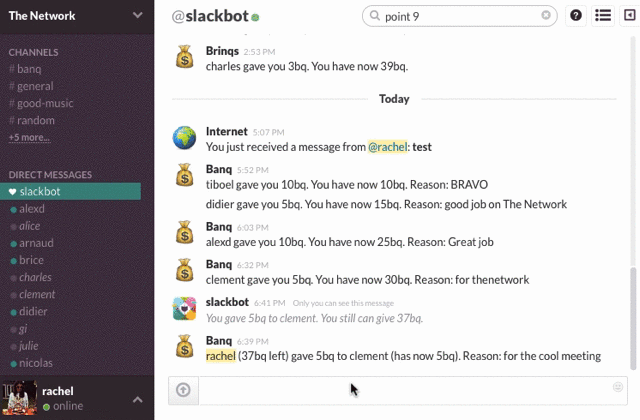
You can follow the transactions on a dedicated webpage and a dedicated Slack channel: information is public so that people self-regulate, do not cheat, and get incentivized to stay on top!
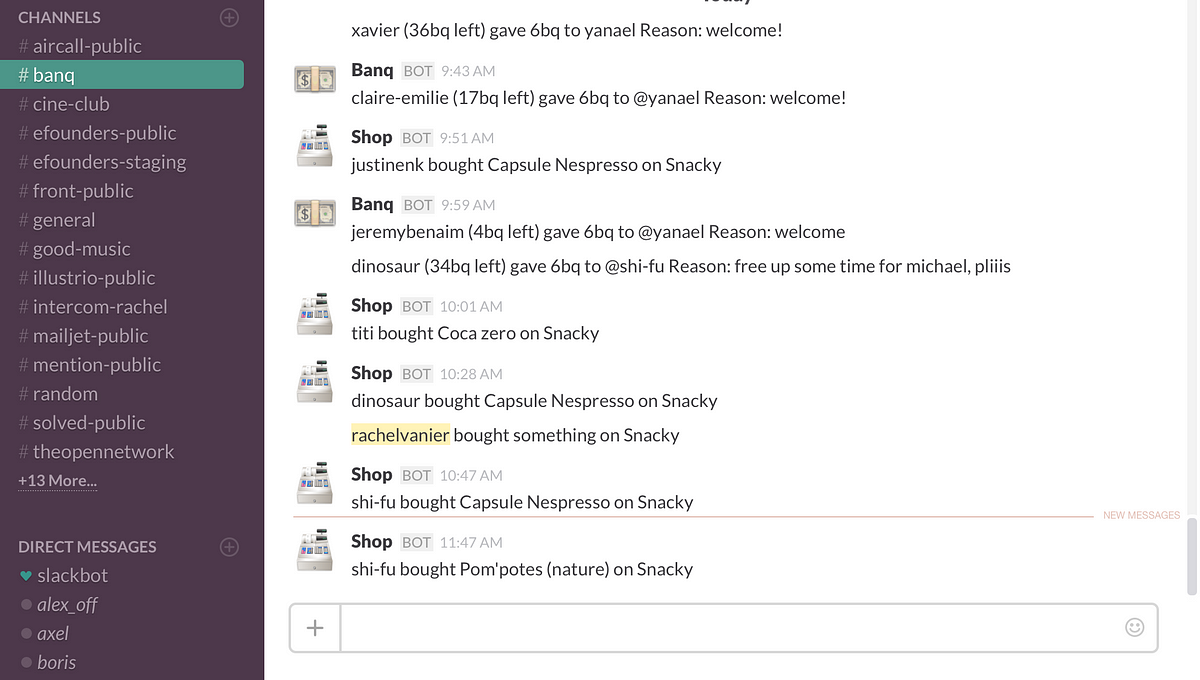
Inside the machine: build your own Briqs
You want to build your own Briqs? Here’s the open source code: https://github.com/efounders/efounders-briqs
In order to develop Banq, we chose to use Meteor. Meteor is platform 100% based on Javascript used to quickly develop distributed applications.
Creating Banq’ users and accesses through Slack’s API
Building the Directory
Before building Banq, we used Slack’s API to build the Directory gathering all the team members of the Network. It’s quite useful when you just joined a company and need to reach out for someone. The Directory is synchronizing with Slack in order to add or modify people’s information.
var result = HTTP.call('GET', 'https://slack.com/api/users.list’, {params: {token: SlackToken}});
“result” contains a JSON with all information on Slack’s users. You just need to add or change the corresponding document in the collection
This function is called every hour to recover possible changes:
Meteor.setInterval(function() {
updateSlackMembers();
}, 1000*60*60);
Authentification
Only members of The Network must be able to edit their own data: we needed to build an authentication system to control access.
Slack provides an OAuth2.0 authentication system (see here). Meteor has a generic authentication system that enables users to login using social networks or simply your email and a password. Consequently, we’ve build a new authentication package on Slack, based on the existing one. We just needed to change URLs and some settings to make it work.
This package is public and free on Github. Feel free to use it!
Building the Briqs
For each user, we need to store 3 values:
- the volume of briqs he can give
- the volume of briqs he received
- the volume of briqs he already gave out
Schemas.Briq = new SimpleSchema({
has: {
type: Number,
defaultValue: 0,
},
canGive: {
type: Number,
defaultValue: 0,
},
gave: {
type: Number,
defaultValue: 0,
}
});
Add a Slack command
First, we need to add a Slack integration called “Slack Commands”, which enables our users to trigger a query on our server using a command directly inside Slack (in our case: /give).
- inside Slack
When adding the Slack Commands integration, you need to configure the name of your command, the URL Slack shall call and some other settings.
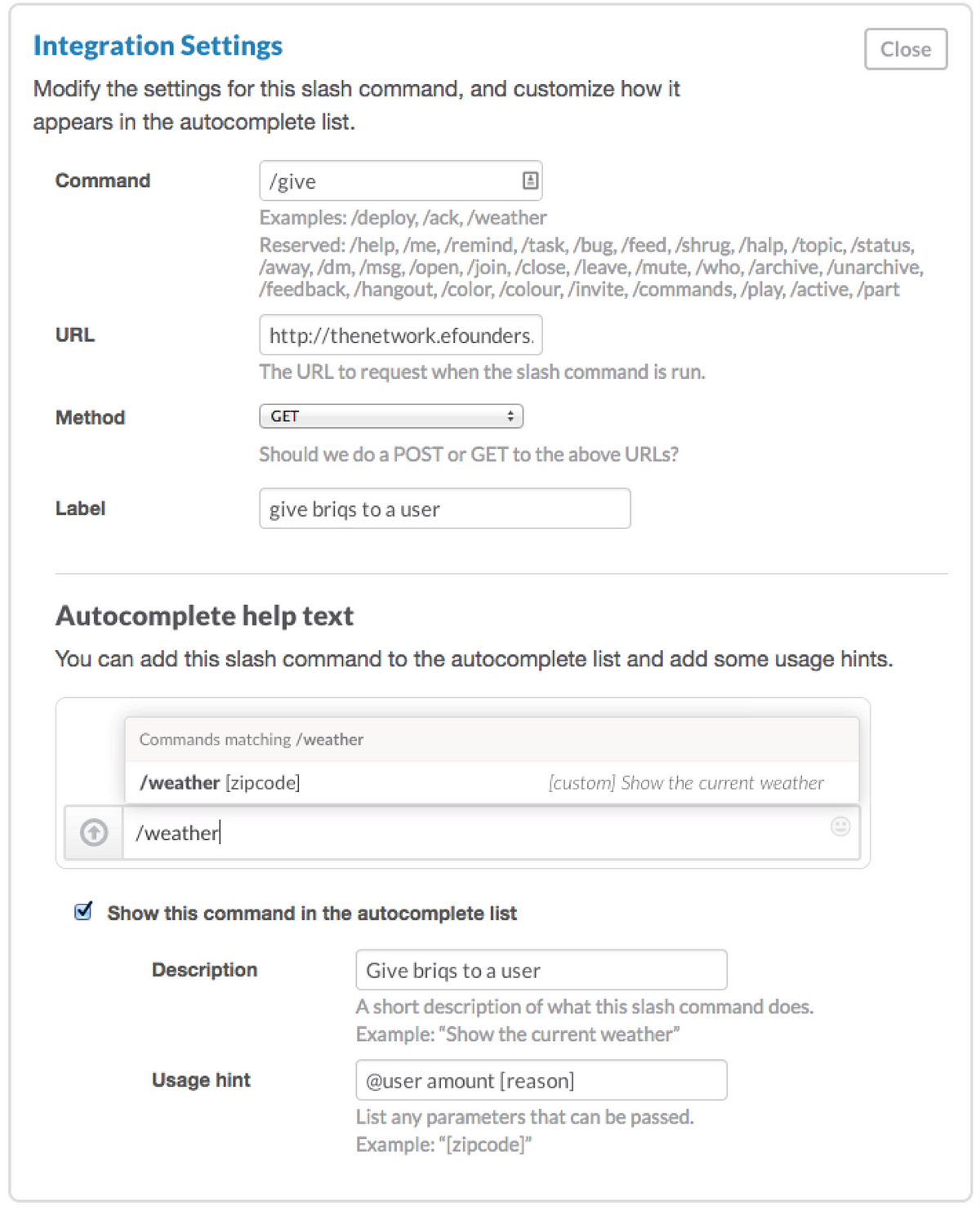
- inside Meteor
Now Meteor has to receive the query sent via Slack. In order to do so, the “iron-router” package is perfect. Although the classical model when you develop single page applications is to do your routing on the client side, iron-router enables you to define server side routes.
Router.map(function() {
this.route('slackCommand/give', {
where: 'server',
action: function() {
if(this.params.token !== <integration_token>) { this.response.end('bad token'); return; }
if(this.params.text === '') { this.response.end('/give <username> <amount> [<reason>]'); return; }
params = this.params.text.split('+');
if(params.length < 2) { this.response.end('/give <username> <amount> [<reason>]'); return; }
...
}
});
});
As you can see in the code, we defined the route name (in our case: ‘slackCommand/give’) then we test the parameters. It is really important to check that the token — which is unique to each integration — is the right one to prevent anyone from trying to execute the command outside Slack.
Once all settings are checked, you just need to execute the command, and start becoming rich (or briqch).
If you have any questions on how we’ve built the Briqs, give us a holler: @eFounders. We’d also love to hear your stories of Slack’s customization!
You wanna play with the Briqs? Come join our team(s)